実行イメージ
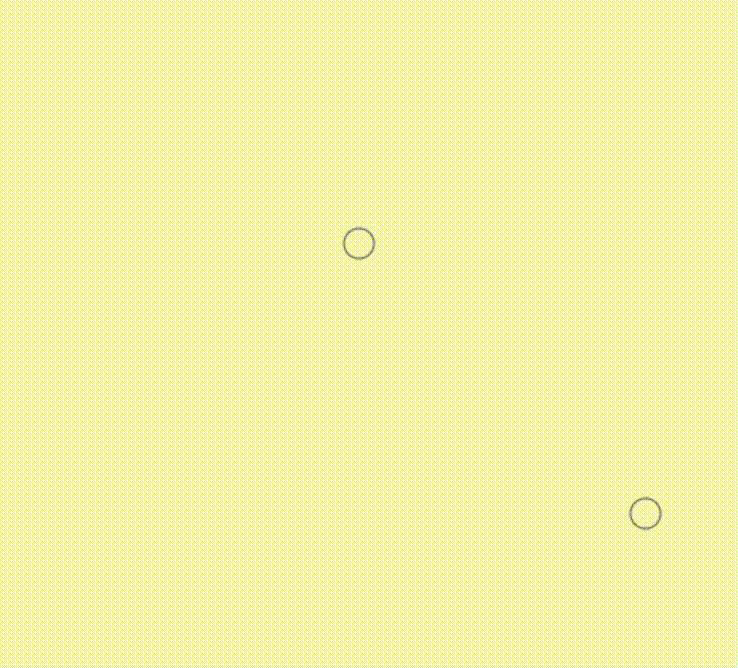
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<script src="Object.js" type="text/javascript"></script>
<script src="main.js" type="text/javascript"></script>
<title>基本のゲームループ描画</title>
</head>
<body>
<canvas id="canvas" width="500" height="500"></canvas>
</body>
</html>
style.css
/* style.css */
@charset "utf-8";
*{
margin: 0;
padding: 0;
}
Object.js
class Object{
// コンストラクタ(x座標, y座標, x方向速度, y方向速度)
constructor(x, y, vx, vy, target){
this.x = x; // x座標
this.y = y; // y座標
this.vx = vx; // x方向速度
this.vy = vy; // y方向速度
this.isAlive = true; // オブジェクトの生死
}
// 移動メソッド
move(){
this.x += this.vx;
this.y += this.vy;
// 画面から消えたら消去
if(this.x < 0 || this.x > canvas.width || this.y < 0 || this.y > canvas.height){
this.isAlive = false;
console.log("消滅");
}
}
// 描画メソッド
draw(){
g.beginPath();
g.strokeStyle = "#555";
g.arc(this.x, this.y, 10, 0, Math.PI*2, true);
g.stroke();
}
// 更新処理
update(){
this.move();
this.draw();
}
}
main.js
// main.js
let canvas = null;
let g = null;
let objects = []; // インスタンス格納用配列
let frameCount = 0; // フレーム数
// 指定範囲の乱数を生成する関数(min~max)
function randInt(min, max){
return Math.floor(Math.random() * (max+1-min)+min);
}
// オブジェクトを消去
function deleteObjects(){
// 画面外は配列を消去
for(let i in objects){
if(!objects[i].isAlive) objects.splice(i, 1);
}
}
// 描画更新処理
function mainLoop(){
// キャンバスクリア
g.fillStyle = "#eee";
g.fillRect(0, 0, canvas.width, canvas.height);
// 一定時間ごとにオブジェクトを生成
if(frameCount % 60 == 0){
// x座標をランダムに設定
const x = randInt(0, canvas.width);
// オブジェクトを生成
const object = new Object(randInt(0, canvas.width), 0, 0, 3);
console.log({object});
// オブジェクトを配列に格納
objects.push(object);
}
// オブジェクトの描画位置を更新
for(let object of objects){
object.update();
}
// オブジェクト消去
deleteObjects();
// フレームカウント
frameCount++;
// フレーム毎に再帰呼び出し
requestAnimationFrame(mainLoop);
}
window.addEventListener("load", ()=>{
// キャンバス取得
canvas = document.getElementById("canvas");
g = canvas.getContext("2d");
frameCount = 0;
// 描画更新処理を開始
mainLoop();
});
コメント