Unity Editor画面
全体
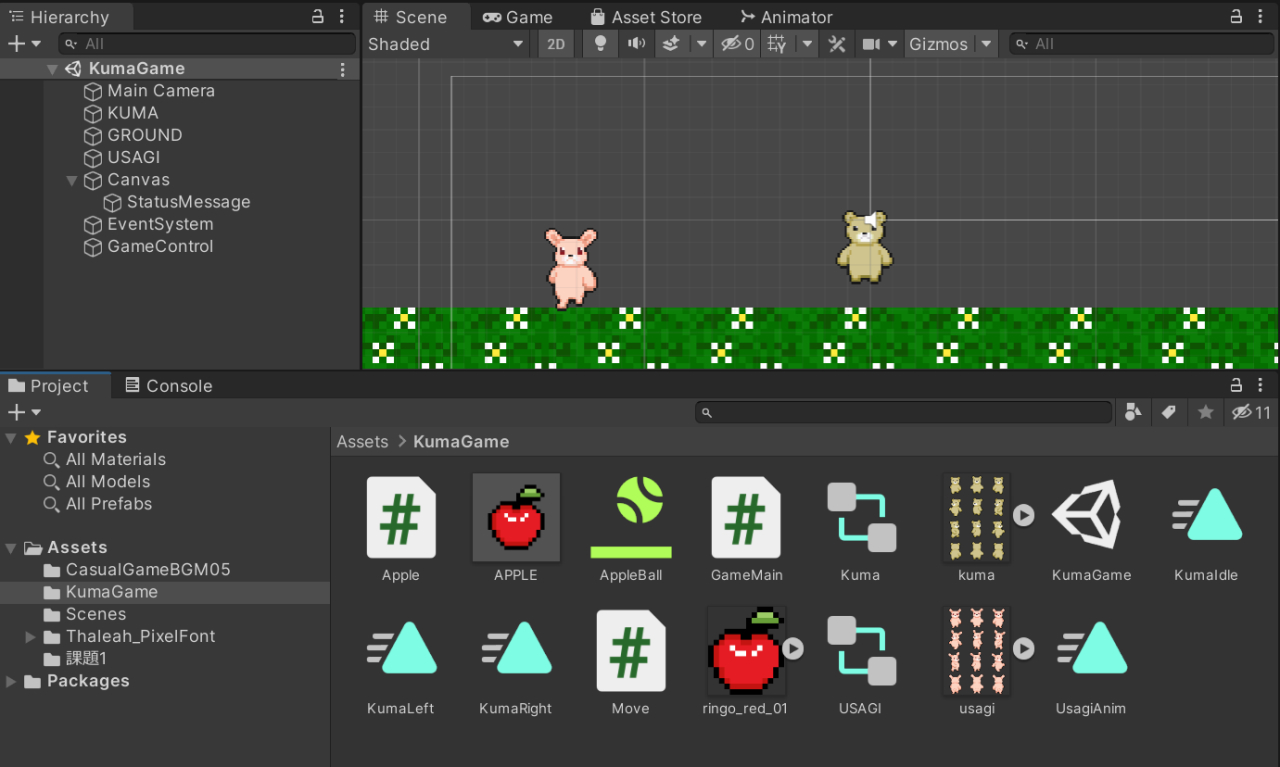
GROUND
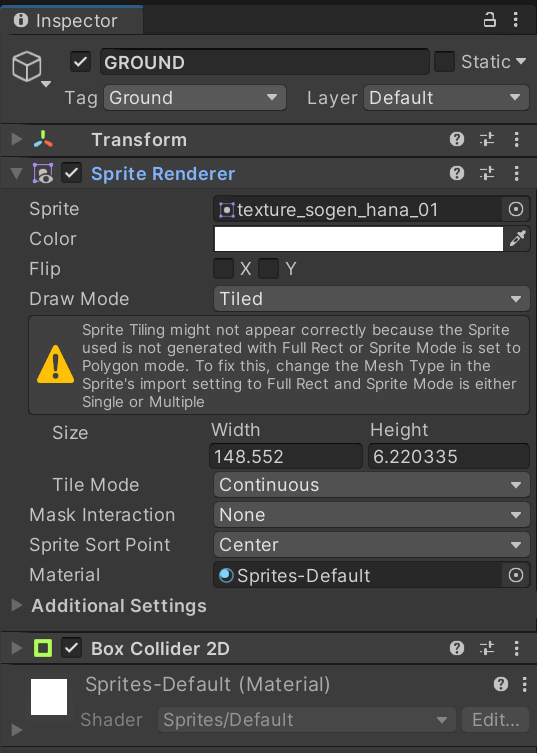
KUMA
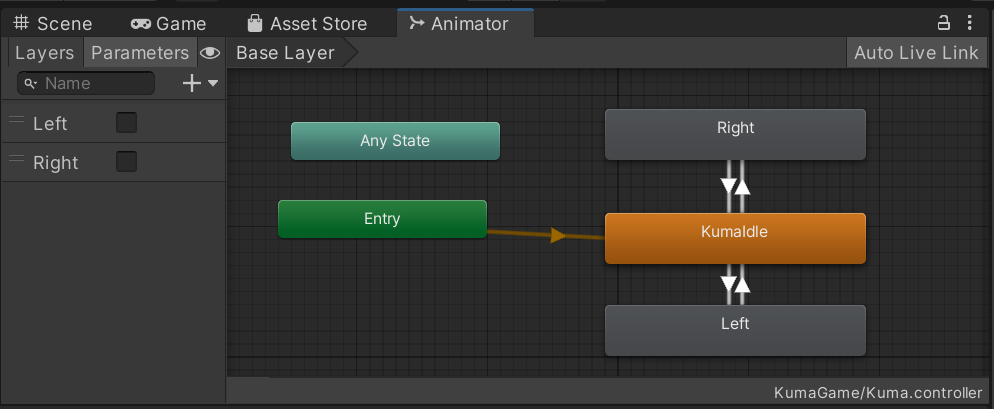
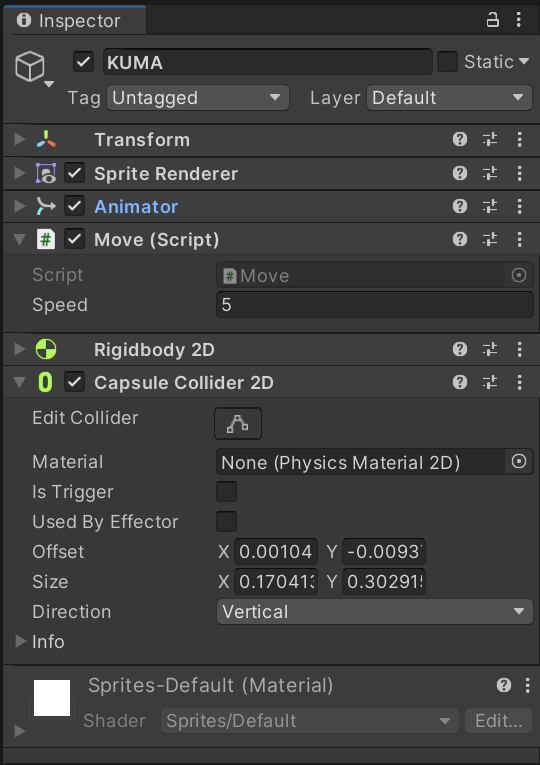
USAGI
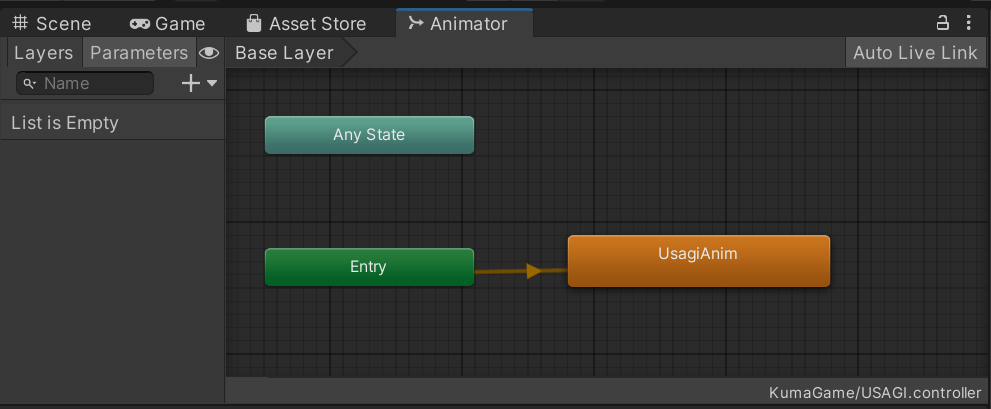
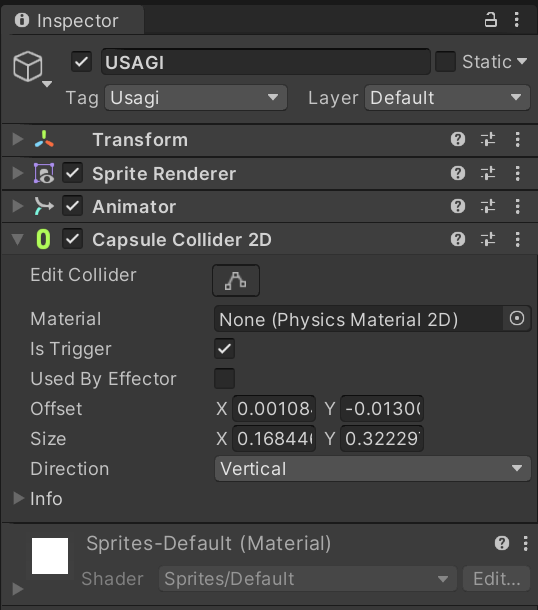
APPLE
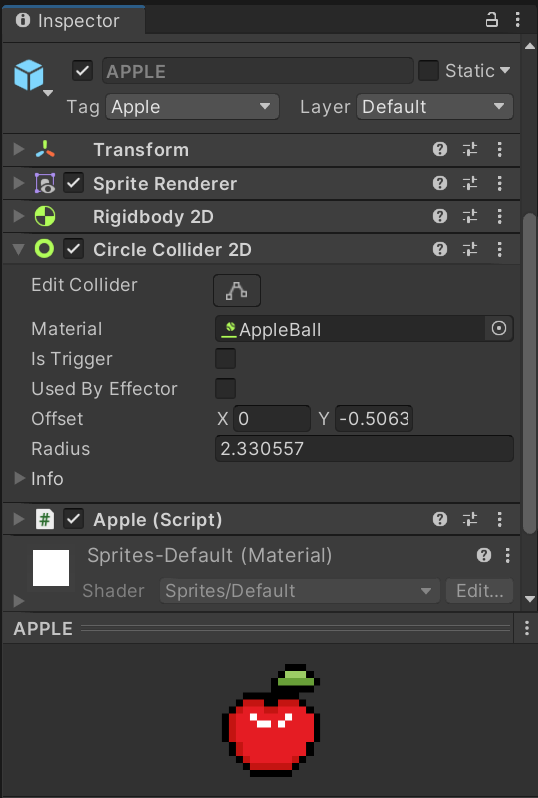
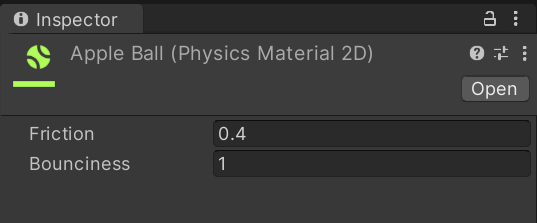
GameControl
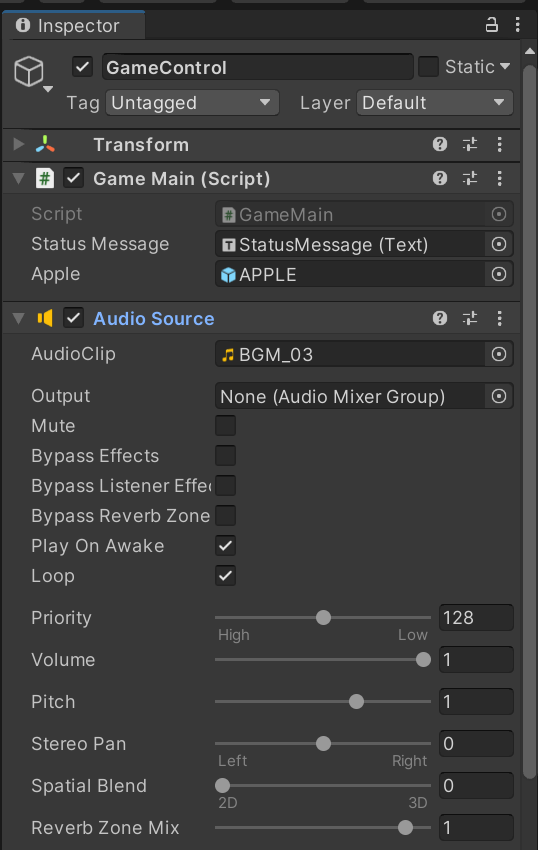
スクリプト
Move
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 | using System.Collections; using System.Collections.Generic; using UnityEngine; public class Move : MonoBehaviour { [SerializeField] float speed = 5.0f; private Animator animator; // Start is called before the first frame update void Start() { animator = GetComponent<Animator>(); } // Update is called once per frame void Update() { if (Input.GetKey(KeyCode.LeftArrow)) { animator.SetBool( "Left" , true ); animator.SetBool( "Right" , false ); transform.position += Vector3.left * speed * Time.deltaTime; } else if (Input.GetKey(KeyCode.RightArrow)) { animator.SetBool( "Right" , true ); animator.SetBool( "Left" , false ); transform.position += Vector3.right * speed * Time.deltaTime; } else { animator.SetBool( "Right" , false ); animator.SetBool( "Left" , false ); } } } |
Apple
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 | using System.Collections; using System.Collections.Generic; using UnityEngine; public class Apple : MonoBehaviour { private enum STATUS { GAME, GAMEOVER } GameObject gameControl; STATUS status; // Start is called before the first frame update void Start() { status = STATUS.GAME; gameControl = GameObject.Find( "GameControl" ); } private void OnCollisionEnter2D(Collision2D collision) { if (collision.gameObject.CompareTag( "Ground" )) { status = STATUS.GAMEOVER; gameControl.GetComponent<GameMain>().GameOver(); } } private void OnTriggerEnter2D(Collider2D collision) { if (status == STATUS.GAME && collision.gameObject.CompareTag( "Usagi" )){ gameControl.GetComponent<GameMain>().GameClear(); Destroy(gameObject, 0.2f); } } } |
GameMain
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | using UnityEngine; using UnityEngine.UI; using UnityEngine.SceneManagement; public class GameMain : MonoBehaviour { public Text StatusMessage; public GameObject apple; public void Start() { Instantiate(apple, new Vector2(Random.Range(-9f, 9f), Random.Range(6f, 8f)), Quaternion.identity); } private void GameStart() { SceneManager.LoadScene( "KumaGame" ); } public void GameClear() { StatusMessage.text = "CLEAR !!" ; Invoke(nameof(GameStart), 5f); } public void GameOver() { StatusMessage.text = "GAME OVER!" ; Invoke(nameof(GameStart), 3f); } } |
コメント