JSDocは、JavaScriptコードに記述したコメントからHTMLドキュメントを自動生成するツールです。
利用条件:Node.jsがインストール済みであること
JSDocのインストール
コマンドプロンプト上で
npm install -g jsdoc
を実行する。
classをドキュメント化する例
以下のようなファイルを例に説明する。
index.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="main.js"></script>
<title>RPGのキャラクタをクラス化してみる</title>
</head>
<body>
<p>コンソールに表示しています...</p>
</body>
</html>
main.js
// main.js
class Character{
constructor(name, hp, mp, type, level){
this.name = name;
this.hp = hp;
this.mp = mp;
this.type = type;
this.level = level;
}
setHp(hp){
this.hp = hp;
}
getType(){
return this.type;
}
getName(){
return this.name;
}
showInfo(){
console.log(`${this.name}`);
console.log(`HP: ${this.hp}`);
console.log(`MP: ${this.mp}`);
console.log(`${this.type}/Level ${this.level}`);
}
}
window.addEventListener("load", ()=>{
// クラスのインスタンス化
const char1 = new Character("レオンハルト", 100, 20,"戦士", 2);
const char2 = new Character("カイア", 200, 0, "魔導士", 3);
// クラスのメソッドを利用
char1.showInfo();
char2.showInfo();
});
上記、main.js内のクラスCharacterをJSDoc用のドキュメント生成方法に則って記述すると以下のようになる。
class部分のみ抜粋
/**
* @classdesc RPGのキャラクタを管理します
* @author Shuichi Takeda
* @version 1.0.0
*/
class Character{
/**
*
* @param {string} name 名前
* @param {int} hp ヒットポイント
* @param {int} mp マジックポイント
* @param {string} type 種族
* @param {int} level レベル
*/
constructor(name, hp, mp, type, level){
this.name = name;
this.hp = hp;
this.mp = mp;
this.type = type;
this.level = level;
}
/**
* HPを設定します
* @param {int} hp 設定するHP
*/
setHp(hp){
this.hp = hp;
}
/**
* 種族を返します
* @return {string} type
*/
getType(){
return this.type;
}
/**
* 名前を返します
* @return {string} name
*/
getName(){
return this.name;
}
/**
* キャラクタ情報(名前、HP、MP、レベル)をコンソールに表示します。
*/
showInfo(){
console.log(`${this.name}`);
console.log(`HP: ${this.hp}`);
console.log(`MP: ${this.mp}`);
console.log(`${this.type}/Level ${this.level}`);
}
}
参考
Documentation - JSDocリファレンス
TypeScriptを備えたJavaScriptはどのようなJSDocをサポートしているか
HTMLドキュメントを生成する
コマンドプロンプトで該当フォルダに移動し、
jsdoc main.js
などのように実行する。
outフォルダが生成される。outフォルダにあるindex.htmlを開くと以下のようなイメージでドキュメントが生成されている。
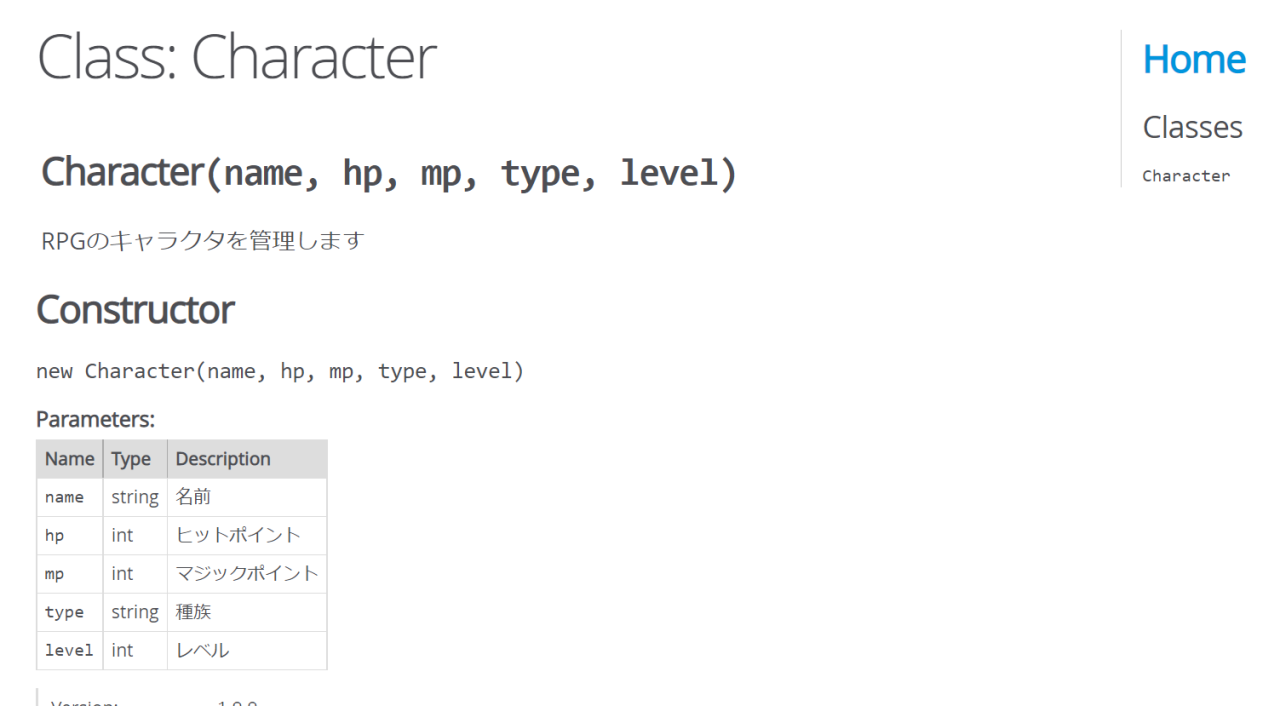
コメント